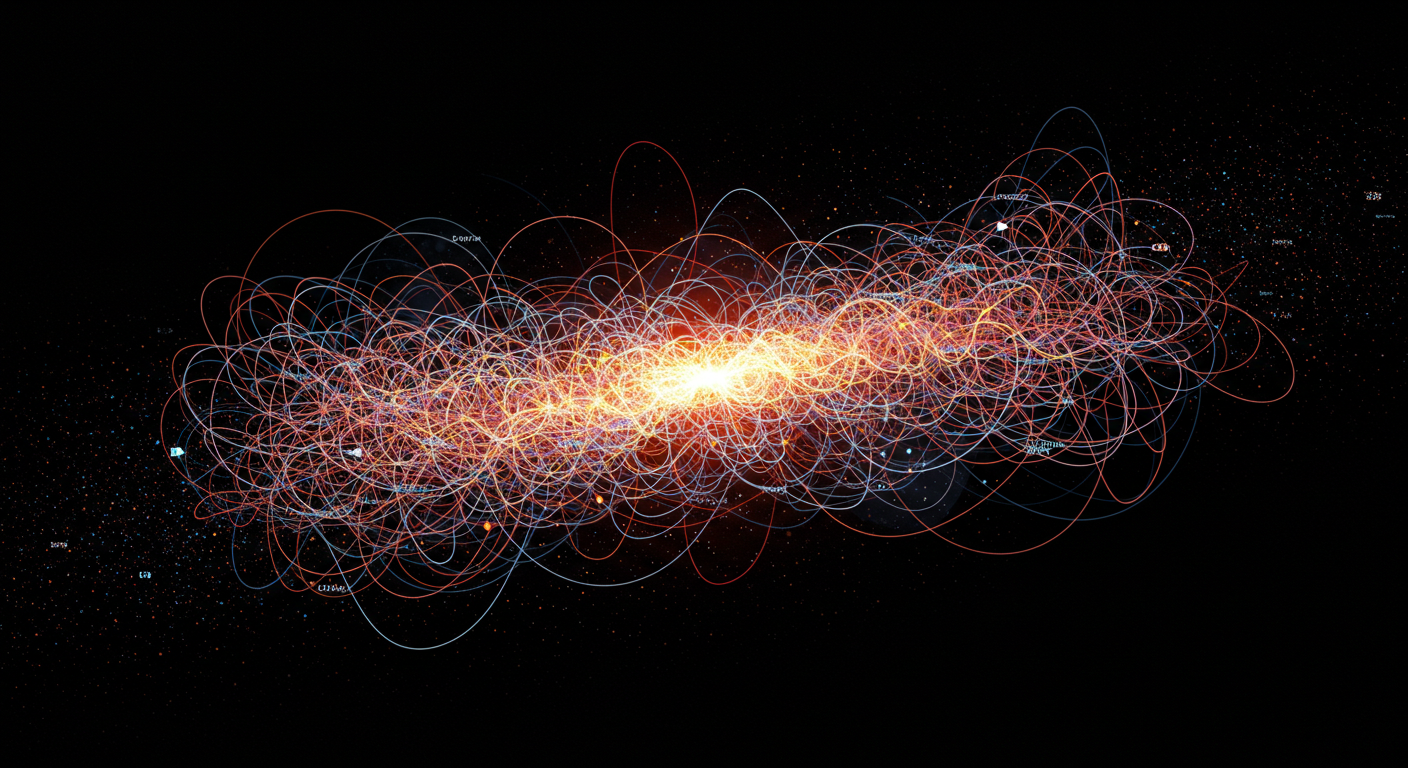
Exploring Async/Await with AI: How I Got a Handle on Asynchronous JavaScript
Exploring Async/Await with AI: How I Got a Handle on Asynchronous JavaScript
Ah, asynchronous JavaScript. If you’ve ever ventured into web development, especially dealing with fetching data, animations, or any operation that takes time, you’ve undoubtedly encountered the need for it. For a long time, my relationship with async/await
was… complicated. I understood the why – to avoid blocking the main thread and keep UIs responsive – but the how and the when sometimes felt like navigating a maze in the dark. Callbacks led to “callback hell,” and while Promises were a huge step up, async/await
promised an even cleaner, more synchronous-looking syntax.
Enter AI coding assistants. I’ve been increasingly using tools like Cursor (an AI-first code editor) and other AI chat interfaces, and I decided to really lean on them to solidify my understanding of async/await
. Today, I want to share how this AI-assisted journey helped me get comfortable with this crucial JavaScript feature.
Why async/await
Even Matters (A Quick Refresher)
Before diving into the AI part, let’s quickly touch on why async/await
is such a big deal. JavaScript is single-threaded, meaning it can only do one thing at a time. If you perform a long-running operation (like fetching data from a remote server) synchronously, your entire webpage freezes until that operation completes. Not a great user experience!
Asynchronous operations allow these long tasks to happen in the background. async/await
is syntactic sugar built on top of Promises, making asynchronous code look and behave a bit more like synchronous code, which many find easier to read and reason about.
My Initial Stumbling Blocks
Even knowing the theory, I’d often find myself:
- Forgetting to mark a function with
async
. - Misunderstanding where
await
could or couldn’t be used. - Getting tangled up in how to properly handle errors in
async
functions. - Struggling to refactor older Promise-based code into the
async/await
style confidently.
It wasn’t a total block, but there was friction. I knew there had to be a smoother way to internalize it.
Bringing in My AI Coding Partner
This is where AI tools like Cursor really started to shine for me. I began treating my AI assistant as a patient tutor and a skilled pair programmer.
Example 1: Getting the Core Concepts Straight
My first step was to go back to basics. I’d ask prompts like:
My Prompt to AI:
“Explain JavaScript async/await
to me as if I’m comfortable with Promises but want to understand the async/await
syntax better. Provide a simple example of fetching data from a public API using async/await
and fetch
.”
A Conceptual AI Response Snippet:
// The AI would explain:
// 1. 'async' keyword: Makes a function return a Promise.
// 2. 'await' keyword: Pauses the execution of an async function until a Promise is settled (resolved or rejected).
// It can only be used inside an 'async' function.
async function fetchData(url) {
try {
// 'await' pauses execution here until fetch() Promise resolves
const response = await fetch(url);
// 'await' pauses execution here until response.json() Promise resolves
const data = await response.json();
console.log(data);
return data; // This will be the resolved value of the Promise returned by fetchData
} catch (error) {
console.error("Failed to fetch data:", error);
// If an error occurs, the Promise returned by fetchData will be rejected with this error
throw error;
}
}
// To use it:
fetchData('https://jsonplaceholder.typicode.com/todos/1');
The AI’s ability to provide clear, commented code examples alongside explanations was incredibly helpful. It wasn’t just theory; it was applied theory.
Example 2: Refactoring Existing Promise Chains
I had some older projects with .then().catch()
chains. I decided to use AI to help refactor them.
My Prompt to AI:
“Can you help me refactor this JavaScript code, which uses Promises with .then()
and .catch()
, into an equivalent version using async/await
? Here’s the original code:
function getUserData(userId) {
fetch(`/api/users/${userId}`)
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
return response.json();
})
.then(data => {
console.log("User data:", data);
return fetch(`/api/users/${userId}/posts`);
})
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
return response.json();
})
.then(postsData => {
console.log("User posts:", postsData);
})
.catch(error => {
console.error("Error fetching user details:", error);
});
}
”
AI’s Refactored Code (Conceptual):
async function getUserDataAsync(userId) {
try {
const userResponse = await fetch(`/api/users/${userId}`);
if (!userResponse.ok) {
throw new Error(`HTTP error! status: ${userResponse.status}`);
}
const userData = await userResponse.json();
console.log("User data:", userData);
const postsResponse = await fetch(`/api/users/${userId}/posts`);
if (!postsResponse.ok) {
throw new Error(`HTTP error! status: ${postsResponse.status}`);
}
const postsData = await postsResponse.json();
console.log("User posts:", postsData);
} catch (error) {
console.error("Error fetching user details:", error);
}
}
Seeing the direct comparison made the benefits of async/await
(especially for sequential asynchronous operations) crystal clear. The code became much flatter and easier to follow.
Example 3: Clarifying Error Handling
This was a big one for me. How does try...catch
interact with await
?
My Prompt to AI:
“Show me the correct way to handle errors in a JavaScript async
function when I’m using await
for multiple operations. What happens if one of the await
ed Promises rejects?”
The AI would then generate an example similar to the try...catch
block in the refactored code above, explaining that await
will propagate rejections, which can then be caught by a standard try...catch
block, just like synchronous code. This demystified error handling significantly.
(Imagine a simple diagram here showing an async function with an await call inside a try block, and an error being caught in the catch block.)
Practical Implementation: Building with AI Assistance
Armed with this clearer understanding, I started applying it to new, small projects. For instance, if I needed to fetch data from three different API endpoints sequentially, where each call depended on the result of the previous one, I’d sketch out the logic and then ask AI:
“I need to write an async
JavaScript function that:
- Fetches user details from
/api/user
. - Uses the user ID from the response to fetch their posts from
/api/posts?userId={id}
. - Then uses the first post ID to fetch comments from
/api/comments?postId={postId}
. Can you help me structure this usingasync/await
and proper error handling?”
The AI would generate a solid starting point, which I could then test, tweak, and integrate. This iterative process of learning, applying with AI help, and then refining was key.
Tips for Using AI to Grok async/await
(or any code concept!)
- Be Specific: Vague questions get vague answers. The more context you provide, the better the AI can help.
- Ask “Why?”: Don’t just accept the code. Ask the AI why it structured something a certain way, or what a particular line does. “Why did you use
Promise.all
here instead of awaiting sequentially?” - Experiment and Break Things: Take the AI’s code, modify it, introduce deliberate errors, and see how it behaves. This is a great way to learn.
- Compare and Contrast: Ask the AI to show you how the same problem would be solved with callbacks, then Promises, then
async/await
. This highlights the evolution and benefits. - Use it for Debugging: If your
async
code isn’t working, paste it and the error (if any) and ask the AI for help debugging.
AI: My Go-To Partner for Untangling Code Knots
Using AI assistants like Cursor as a learning partner for async/await
has been a game-changer for me. It’s like having an infinitely patient senior developer available 24/7 to explain concepts, refactor code, and help troubleshoot. The barrier to understanding complex topics feels much lower.
Of course, it’s not a replacement for fundamental learning and practice, but it’s an incredible accelerator. I’m no longer hesitant when I see async
or await
; instead, I feel equipped to use them effectively, thanks in no small part to my AI coding companions.
What are your experiences using AI tools to learn tricky coding concepts? Have you used them for async/await
or other JavaScript features? I’d love to hear your thoughts and recommendations in the comments!