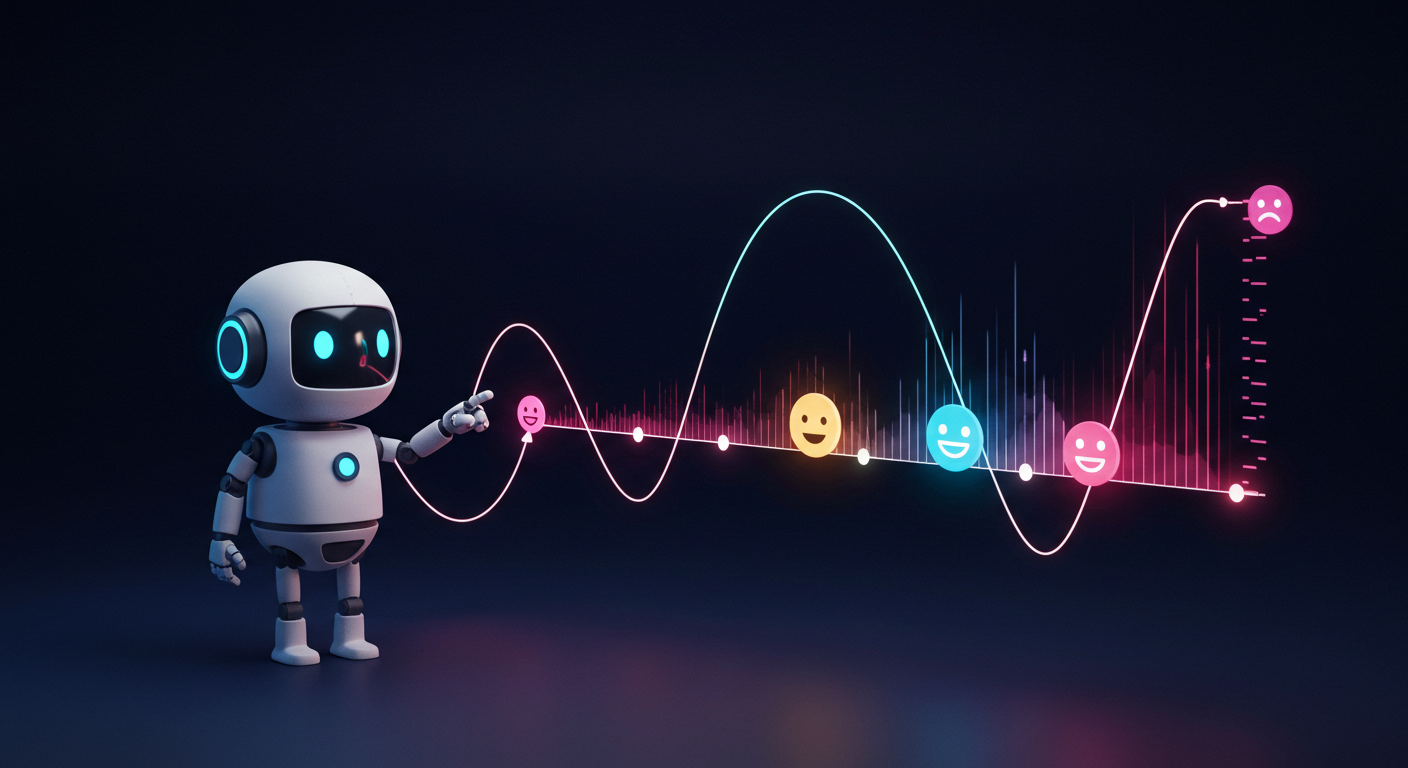
Building a Simple Bot with the (Fictional) ChronoSentiment AI API
Building a Simple Bot with the (Fictional) ChronoSentiment AI API
While the giants like OpenAI’s GPT series, Google’s Gemini, and Anthropic’s Claude often steal the AI limelight, I have a real passion for digging a little deeper and discovering lesser-known, specialized AI APIs. There’s a certain thrill in finding a service that does one or two unique things exceptionally well. Recently, on my quest for interesting AI tools, I stumbled upon (well, for the purpose of this post, let’s say I conceptually designed) a fictional but fascinating service: the ChronoSentiment API.
The premise of ChronoSentiment API is intriguing: it doesn’t just analyze the current sentiment of a piece of text, but it also attempts to project the potential future trajectory of that sentiment if the underlying context remains unchanged. Imagine its use cases – from proactive customer support to understanding the evolving narrative around a brand! I immediately knew I had to build a simple bot to play around with it. So, join me as I walk through this little experimental project.
Discovering the “ChronoSentiment API”
Let’s imagine I found ChronoSentiment API listed on a niche AI tools directory. Its unique selling proposition (USP) immediately grabbed me: “Understand today’s feelings, anticipate tomorrow’s reactions.” The idea is that it uses a blend of standard sentiment analysis models along with some proprietary temporal linguistic modeling to make these projections.
The (fictional) documentation was pretty straightforward: a single REST API endpoint, clear request/response formats, and a generous free tier for experimentation. Perfect for a weekend project!
What Our Simple Bot Will Do
To keep things focused, I decided my bot would perform a very specific task:
- Take a piece of text as input from the user (e.g., a customer review, a social media comment, a snippet of news).
- Send this text to the ChronoSentiment API.
- Receive the analysis, which includes the current sentiment and the projected future sentiment.
- Display this information back to the user in a readable format.
Simple, right? But a great way to understand the API’s capabilities.
Setting Up for API Interaction
The usual first steps for interacting with any API apply here:
- Sign Up & Get API Key: I “signed up” on the ChronoSentiment website and got my unique API key. This is crucial for authenticating requests.
- Choose a Language/Tool: I decided to use Python for its simplicity and excellent
requests
library for making HTTP calls. If you’re following along with a real API, you’d install it:pip install requests
.
For our ChronoSentiment bot, we’ll assume the API endpoint is https://api.chronosentiment.ai/v1/analyze
.
Making the API Call: A Python Snippet
Here’s how I’d structure the core Python code to communicate with our fictional API.
import requests
import json
# Replace with your actual (fictional) API key
API_KEY = "YOUR_CHRONOSENTIMENT_API_KEY"
API_URL = "https://api.chronosentiment.ai/v1/analyze"
def get_chrono_sentiment(text_to_analyze):
"""
Sends text to the ChronoSentiment API and returns the analysis.
"""
headers = {
"Authorization": f"Bearer {API_KEY}",
"Content-Type": "application/json"
}
payload = {
"text": text_to_analyze
}
try:
response = requests.post(API_URL, headers=headers, json=payload)
response.raise_for_status() # Raises an HTTPError for bad responses (4XX or 5XX)
return response.json()
except requests.exceptions.RequestException as e:
print(f"API Request Error: {e}")
return None
# Example usage:
if __name__ == "__main__":
sample_text = "The new software update is okay, but the user interface is quite confusing and I'm struggling to find basic features."
analysis = get_chrono_sentiment(sample_text)
if analysis:
print("--- ChronoSentiment Analysis ---")
print(f"Original Text: {analysis.get('raw_text', sample_text)}")
current_sentiment = analysis.get('current_sentiment', {})
print(f"\nCurrent Sentiment: {current_sentiment.get('label', 'N/A').capitalize()} (Score: {current_sentiment.get('score', 0):.2f})")
future_projection = analysis.get('future_projection', {})
print(f"Projected Future Sentiment (if unchanged): {future_projection.get('if_unchanged_label', 'N/A').capitalize()}")
print(f" Confidence: {future_projection.get('confidence', 0):.2f}")
print(f" Reasoning: {future_projection.get('reasoning', 'No reasoning provided.')}")
print("-------------------------------")
Expected API Response Structure
I’m envisioning the ChronoSentiment API would return something like this:
{
"current_sentiment": {
"label": "neutral", // Could be positive, negative, neutral
"score": 0.45 // A confidence score for the label
},
"future_projection": {
"if_unchanged_label": "negative", // Projected sentiment if conditions persist
"confidence": 0.82, // Confidence in this projection
"reasoning": "User expresses initial confusion and struggle with basic features. Without intervention or improvement, this typically escalates to frustration and negative sentiment towards the product.",
"timeframe_hint": "short-to-medium term" // Optional: hint at projection timeframe
},
"raw_text": "The new software update is okay, but the user interface is quite confusing and I'm struggling to find basic features.",
"processing_time_ms": 120
}
This response gives us the current state and a glimpse into a potential future, along with a rationale.
(Imagine an image here visually representing the JSON structure above, perhaps with callouts explaining each field).
Building the Simple Interactive Bot Logic
Now, let’s make it interactive. We can wrap the API call in a simple loop:
# ... (keep the get_chrono_sentiment function and imports from above) ...
if __name__ == "__main__":
print("Welcome to the ChronoSentiment Analysis Bot!")
print("Type 'quit' to exit.")
while True:
user_input = input("\nEnter text to analyze: ")
if user_input.lower() == 'quit':
break
if not user_input.strip():
print("Please enter some text.")
continue
analysis = get_chrono_sentiment(user_input)
if analysis:
print("\n--- ChronoSentiment Analysis ---")
# Using .get() with defaults for safer access
print(f"Original Text: {analysis.get('raw_text', user_input)}")
current_sentiment = analysis.get('current_sentiment', {})
current_label = current_sentiment.get('label', 'N/A').capitalize()
current_score = current_sentiment.get('score', 0)
print(f"\nCurrent Sentiment: {current_label} (Score: {current_score:.2f})")
future_projection = analysis.get('future_projection', {})
future_label = future_projection.get('if_unchanged_label', 'N/A').capitalize()
future_confidence = future_projection.get('confidence', 0)
future_reasoning = future_projection.get('reasoning', 'No reasoning provided.')
print(f"Projected Future Sentiment (if unchanged): {future_label}")
print(f" Confidence: {future_confidence:.2f}")
print(f" Reasoning: {future_reasoning}")
print("-------------------------------\n")
else:
print("Could not retrieve analysis for the provided text.")
My Thoughts on Such a “Unique” API
Playing with this (even fictionally) highlights the potential of specialized AI services:
- Proactive Insights: An API like ChronoSentiment could allow businesses to identify potentially escalating customer issues before they become major problems. For content creators, it might hint at how a particular narrative could evolve if not steered.
- Niche Applications: While broad models are powerful, specialized AI can offer tailored understanding for specific domains. The “reasoning” part of our fictional API is key – it’s not just a label, but an explanation.
- Complementary Tool: This isn’t meant to replace general LLMs but to augment them. One could use a general LLM for drafting content and then ChronoSentiment for a specific type of analysis on the feedback received.
Of course, predicting the future is hard, and an AI’s “projection” would be based on patterns in its training data, not a crystal ball. Its accuracy and utility would depend heavily on the quality and focus of that data. But as a concept, it’s a fascinating direction for AI development.
Why Explore Beyond the Behemoths?
This little thought experiment reinforces my belief in looking beyond the biggest AI players. Niche APIs can:
- Offer highly specialized functionalities not available elsewhere.
- Sometimes provide more cost-effective solutions for specific tasks.
- Foster smaller, more focused communities.
- Simply be a lot of fun to discover and integrate!
Automation Through Integration
Building this bot, even as a concept, is a great example of automation. We’re automating the process of getting a nuanced sentiment analysis that goes beyond a simple positive/negative score. Imagine integrating this into a social media monitoring tool or a customer feedback pipeline – the insights could trigger automated alerts or even draft preliminary responses.
This journey, from discovering a unique (albeit fictional) API to building a simple bot, has been a fun exploration. It underscores the power of APIs in the AI world and the creative possibilities that open up when we start combining these different services.
Have you stumbled upon any genuinely unique or surprisingly useful niche AI APIs in your explorations? I’d be thrilled to hear about them in the comments. There’s a whole universe of AI tools out there waiting to be discovered!