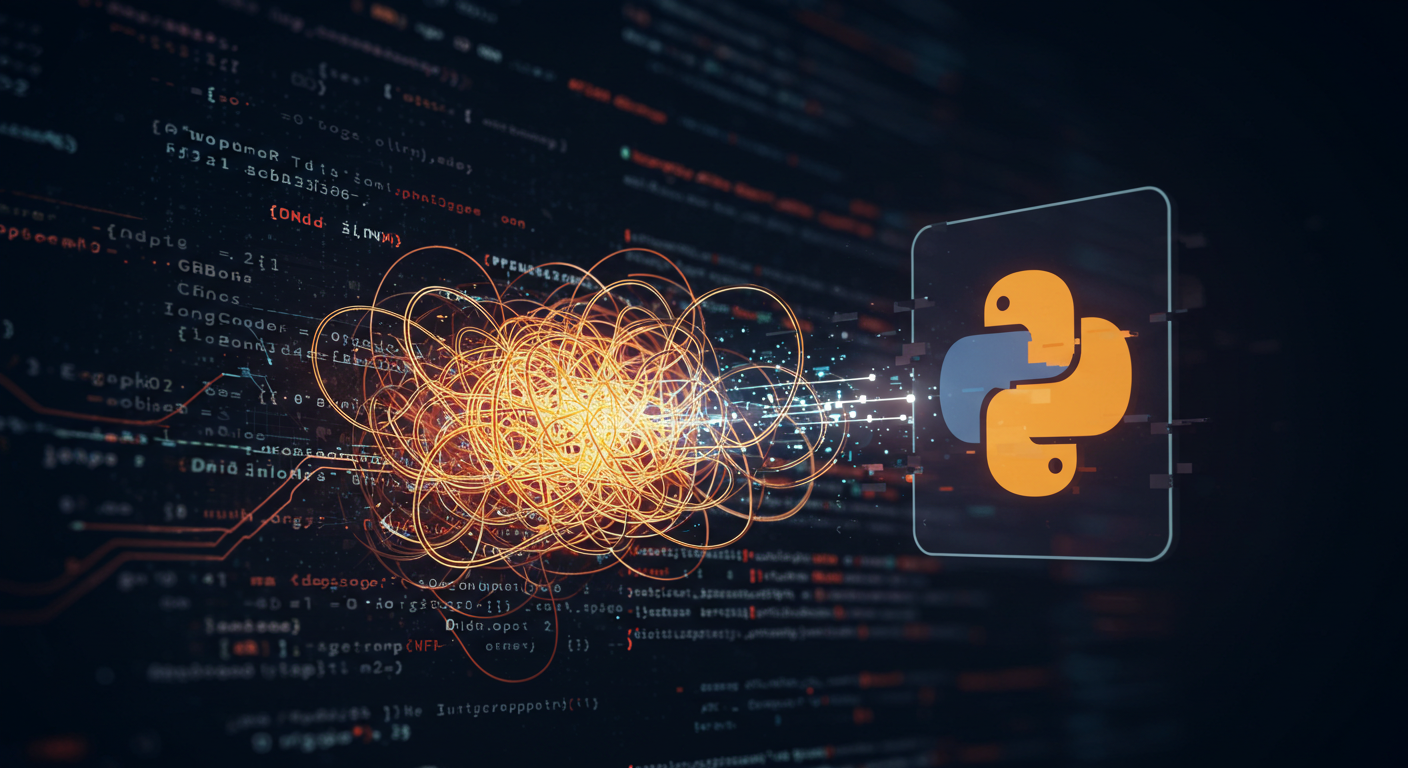
Debugging Python with AI: My New Favorite Coding Buddy
Debugging Python with AI: My New Favorite Coding Buddy
Ah, debugging. That beloved pastime of every developer. You know the drill: you’ve written what you think is perfectly good Python code, you hit “run,” and then… TypeError
, IndexError
, or the dreaded silent failure where your logic just isn’t doing what you expect. For years, my debugging toolkit consisted of print()
statements, a trusty debugger like pdb
, and a lot of head-scratching. But recently, I’ve added a powerful new ally to my arsenal: AI assistants!
I was initially a bit skeptical. Could an AI really understand my messy, half-finished code and pinpoint the elusive bug? The answer, I’ve found, is a resounding “Yes, often surprisingly well!” Today, I want to share how I’ve been using AI tools like ChatGPT, GitHub Copilot Chat, and others to make my Python debugging process faster, more insightful, and even a bit more fun.
Why AI for Debugging? (The “Aha!” Moment)
My “aha!” moment came when I was stuck on a particularly tricky bug in a data processing script. I’d been staring at it for an hour, getting nowhere. On a whim, I pasted the problematic function and the traceback into an AI chat interface. Within seconds, it not only pointed out a subtle off-by-one error I’d completely missed but also explained why it was happening in the context of my code.
Here’s why I think AI is becoming invaluable for debugging:
- Speed: AI can often spot syntax errors or common logical flaws much faster than I can by manually tracing.
- A Fresh Perspective: It’s like having an infinitely patient pair programmer who hasn’t been staring at the same code for hours. It can see things your tired brain might overlook.
- Learning Opportunity: AI doesn’t just fix the bug; it often explains the underlying concept, helping me learn and avoid similar mistakes in the future.
- The “Rubber Ducky” on Steroids: Sometimes, just the act of formulating your problem clearly for the AI helps you spot the issue yourself. But unlike a traditional rubber duck, this one talks back with useful suggestions!
Getting Started: Preparing Your Code and Prompt for the AI
To get the most out of AI for debugging, you can’t just dump your entire codebase and say “fix it.” Here’s how I prepare:
- Provide Context: Briefly explain what the code is supposed to do. What’s its objective? This helps the AI understand intent.
- Isolate the Problem: If possible, provide the smallest snippet of code that reproduces the bug. This makes it easier for the AI to focus. If it’s a larger function, indicate the specific lines you suspect.
- Share the Full Error Message: Copy and paste the complete traceback. This is packed with clues (file names, line numbers, error types) that the AI can use.
- Mention Your Environment (if relevant): Sometimes, your Python version, operating system, or specific libraries and their versions can be crucial. E.g., “I’m using Python 3.9 on Windows with Pandas 1.5.0.”
Smart Prompting Strategies for Debugging
The quality of your prompt significantly impacts the AI’s response. Here are some of my go-to prompts:
- “Explain this Python error message to me:
[paste traceback here]
”- Great for cryptic errors. The AI can break down what each part of the traceback means.
- “What are common causes for a
[SpecificErrorType, e.g., KeyError]
in Python when working with dictionaries?”- Good for understanding the general nature of an error.
- “Can you spot any potential issues in this Python code snippet that might cause
[describe the problem or paste error]
?”# My problematic code snippet def process_data(data_list): # ... some code ... result = data_list[len(data_list)] # Potential IndexError here! # ... more code ... return result
- Followed by: “The error is
IndexError: list index out of range
.”
- Followed by: “The error is
- “This Python function is supposed to
[expected behavior]
, but it’s[actual, buggy behavior]
. Can you help me find the bug? Here’s the code:[paste code]
”- Useful for logic errors where the code runs but produces the wrong output.
- “Suggest alternative ways to write this Python function to achieve
[desired outcome]
if you think my current approach is flawed.”- Sometimes, the bug is a symptom of a deeper design issue.
(Imagine a screenshot here showing a chat interface with a user pasting Python code and an error, and the AI responding with a suggestion or explanation.)
Common Pitfalls and How to Avoid Them
AI is powerful, but it’s not infallible. Here are some traps to watch out for:
- Blindly Trusting the AI: Always understand the AI’s suggestion before implementing it. Test the fix thoroughly. Sometimes AI suggestions can be subtly wrong or introduce new issues.
- Giving Too Little Context: If you just paste an error without code, or code without the error or intent, the AI is guessing.
- Giving Too Much Code: Pasting thousands of lines of code will likely confuse the AI or lead to very generic advice. Isolate the issue.
- Not Iterating: The first suggestion might not be perfect. Refine your prompt, provide more information, or ask follow-up questions. “That didn’t quite fix it, but now I’m getting this new error…”
Beyond Error Messages: AI for Logic Bugs
AI isn’t just for code that crashes. It can also be surprisingly helpful for those insidious logic bugs where your Python code runs without errors but produces the wrong results.
For these, I typically:
- Clearly describe the expected input and output.
- Provide the actual (incorrect) output.
- Share the relevant function or code block.
- Ask the AI to review the logic for specific conditions or to trace how the input might lead to the incorrect output.
For instance: “My Python function calculate_discount(price, percentage)
should return the price after a discount. If I call calculate_discount(100, 10)
, I expect 90
, but I’m getting 10
. Here’s the code: [def calculate_discount(price, percentage): return price * (percentage / 100)]
. What’s wrong?” (The AI should spot that it’s returning the discount amount, not the final price).
My Personal Workflow: A Quick Example
- Encounter Bug: My Python script throws an unexpected
ValueError
when processing a specific CSV file. - Isolate & Copy: I identify the function handling CSV parsing and copy it along with the full traceback.
- Prompt AI: I open my AI assistant (e.g., GitHub Copilot Chat directly in VS Code, or ChatGPT in a browser) and use a prompt like: “I’m getting a
ValueError: invalid literal for int() with base 10: 'N/A'
with this Python code when processing a CSV. The ‘N/A’ values should probably be treated as 0 or skipped. How can I modify theprocess_row
function to handle this gracefully? Code:[paste function]
Error:[paste traceback]
” - Review & Refine: The AI suggests using a
try-except
block to catch theValueError
duringint()
conversion or to check for ‘N/A’ strings before conversion. - Test & Iterate: I implement the suggestion, test it, and if it works, great! If not, I might ask a follow-up: “Thanks, that handled ‘N/A’, but what if the column is empty? How do I handle that too?”
AI: Your Debugging Supercharger, Not a Replacement
Using AI for debugging has genuinely made me a more efficient Python developer. It helps me get unstuck faster, learn new patterns, and focus more on building features rather than chasing down syntax errors.
However, it’s crucial to remember that AI is a tool. It augments your skills; it doesn’t replace the need for understanding fundamental programming concepts and debugging principles. Always strive to understand why the AI’s suggestion works.
So, if you haven’t already, I highly encourage you to start experimenting with AI assistants in your Python debugging workflow. You might just find your new favorite coding buddy!
What are your experiences using AI for debugging? Any favorite prompts or techniques you’d like to share? Drop a comment below – I’m always eager to learn new tricks!