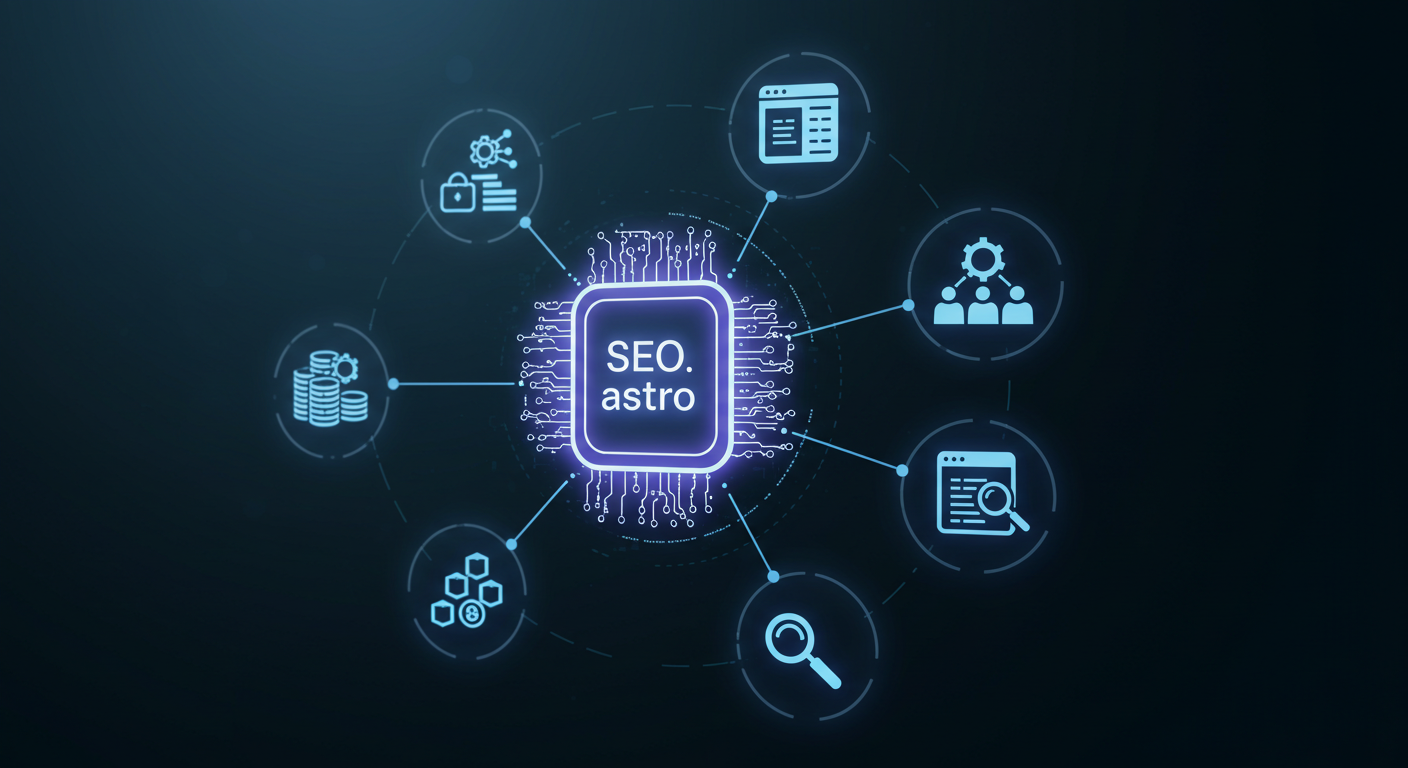
My Quest for Perfect SEO: Building a Reusable SEO Component in Astro
My Quest for Perfect SEO: Building a Reusable SEO Component in Astro
As I’ve been building out Domdhi.com, my personal AI Hub, one thing has been consistently on my mind: Search Engine Optimization (SEO). It’s great to share my AI journey, discoveries, and practical experiences, but what good is it if no one can find the content? I knew from the start that robust SEO wouldn’t be an afterthought; it needed to be baked into the very fabric of the site.
My goal was to ensure every page, whether it’s a blog post about a new AI tool, a coding tutorial, or a journal entry, has all the necessary meta tags for search engines and social media sharing. Doing this manually on every page? No thank you! That’s a recipe for inconsistency and forgotten tags. This led me down the path of creating a powerful, reusable SEO.astro
component. Today, I want to share what this component does, how it works, and why it’s become a cornerstone of my site’s architecture.
Why a Dedicated SEO Component?
Before diving into the component itself, let’s talk about why this is so important:
- Consistency: Ensures every page has the same set of critical SEO tags, formatted correctly.
- Maintainability: If SEO best practices change or I want to add a new meta tag (like for a new social platform), I only need to update it in one place.
- Completeness: It’s easy to forget tags. A component acts as a checklist, ensuring we cover titles, descriptions, canonical URLs, Open Graph data, Twitter Cards, structured data, and more.
- Developer Experience: It makes adding SEO to new pages incredibly simple. Just drop in the component and pass the necessary props.
For a site built with Astro, leveraging its component-based architecture for SEO just makes perfect sense.
Features of My SEO.astro
Component
I designed the SEO.astro
component to be a comprehensive solution. Here’s a rundown of what it handles:
- Core SEO Meta Tags: Generates the fundamental
<title>
and<meta name="description">
tags. - Canonical URLs: Specifies the preferred version of a webpage to avoid duplicate content issues.
- Open Graph Meta Tags: Essential for rich sharing previews on platforms like Facebook and LinkedIn (e.g.,
og:title
,og:description
,og:image
,og:type
). - Twitter Card Meta Tags: Ensures tweets linking to my content look great with summary cards (e.g.,
twitter:card
,twitter:title
,twitter:description
,twitter:image
). - Structured Data (JSON-LD): This is a big one for rich results in search engines! It allows me to describe my content in a way search engines can easily understand, potentially leading to enhanced search listings (like article snippets with publish dates, authors, etc.).
- Configurable Robot Directives: Provides options to tell search engine crawlers
noindex
(don’t index this page) ornofollow
(don’t follow links on this page) when needed. - Article Metadata Best Practices: Includes specific tags beneficial for articles, such as
article:published_time
,article:modified_time
,article:author
, andarticle:tag
. - Dynamic Image URL Handling: Properly formats image URLs for Open Graph and Twitter Cards, ensuring they are absolute URLs.
How I Use the SEO.astro
Component
The beauty of an Astro component is its reusability. I primarily use the SEO.astro
component within my layout components, especially in BaseLayout.astro
which wraps most of my pages. It sits right in the <head>
section.
Basic Usage Example
For a simple static page, the usage might look like this in the page’s frontmatter or layout:
---
// In a layout or page component
import SEO from '../components/SEO.astro'; // Adjust path as needed
---
<head>
{/* Other head elements like charset, viewport */}
<SEO
title="My Awesome Page Title | Domdhi.com"
description="A concise and compelling description of this awesome page for search engines and social media sharing."
/>
{/* Other links, scripts */}
</head>
Advanced Usage for a Blog Post
For blog posts, I leverage more of its capabilities, especially structured data:
---
// In my blog post layout or directly in a blog post .astro file
import SEO from '../components/SEO.astro';
import { generateBlogPostSchema } from '../utils/schema'; // A helper function to create JSON-LD
const { frontmatter } = Astro.props; // Assuming frontmatter contains post details
// Generate structured data for the current blog post
const schema = generateBlogPostSchema({
title: frontmatter.title,
description: frontmatter.description,
image: Astro.url.origin + (frontmatter.heroImage || '/default-og-image.png'), // Ensure absolute URL
publishDate: new Date(frontmatter.date),
modifiedDate: frontmatter.updatedDate ? new Date(frontmatter.updatedDate) : new Date(frontmatter.date),
authorName: frontmatter.author || "Domdhi", // Default author
url: Astro.url.href, // Full URL of the blog post
tags: frontmatter.tags || []
// ... other required properties for my schema generator
});
---
<head>
<SEO
title={`${frontmatter.title} | Domdhi.com`}
description={frontmatter.description}
image={frontmatter.heroImage || "/images/domdhi-og-default.png"} // Site-wide default OG image
type="article"
author={frontmatter.author || "Domdhi"}
publishDate={new Date(frontmatter.date)}
modifiedDate={frontmatter.updatedDate ? new Date(frontmatter.updatedDate) : new Date(frontmatter.date)}
tags={frontmatter.tags}
structuredData={JSON.stringify(schema)} // Pass the stringified JSON-LD
canonical={Astro.url.href} // Explicitly set canonical URL
/>
</head>
(Note: The generateBlogPostSchema
is a conceptual utility function I’d create to keep my structured data generation clean and reusable.)
Available Props for SEO.astro
To make it flexible, the component accepts several props:
Prop | Type | Required | Default | Description |
---|---|---|---|---|
title | string | Yes | - | The page title. Used for <title> and Open Graph/Twitter Card. |
description | string | Yes | - | The page description. Used for meta description and Open Graph/Twitter Card. |
canonical | string | No | Astro.url.pathname | The canonical URL for the page. If not provided, the current URL pathname is used (consider making this Astro.url.href for full URL). |
image | string | No | /og-image.png | The image used for Open Graph/Twitter Card (relative to domain). I ensure it becomes absolute. |
imageAlt | string | No | Same as title | The alt text for the Open Graph/Twitter Card image. |
type | "website" | "article" | "profile" | No | "website" | The Open Graph type. |
author | string | No | "Domdhi" | The author of the content (mainly for articles). |
publishDate | Date | No | - | The date the content was published (mainly for articles). |
modifiedDate | Date | No | - | The date the content was last modified (mainly for articles). |
tags | string[] | No | [] | Tags or keywords for the content (mainly for articles, used for article:tag ). |
noindex | boolean | No | false | Whether to prevent search engines from indexing the page. |
nofollow | boolean | No | false | Whether to prevent search engines from following links on the page. |
structuredData | string | object | No | - | JSON-LD structured data for the page (can pass as object, component stringifies). |
(Self-correction: The original docs said Astro.url.pathname
for default canonical. I’d prefer Astro.url.href
for the full absolute URL or ensure the component constructs it.)
Integration with Site Layouts
As mentioned, the SEO.astro
component is a key part of my BaseHead.astro
component, which is included in all my main layouts. This ensures global <head>
elements and SEO tags are applied consistently.
Conceptual BaseHead.astro
Snippet:
---
// src/components/BaseHead.astro
import "../styles/base.css"; // My global styles
import SEO from "./SEO.astro"; // The star of the show!
// ...other global imports like fonts
interface Props {
title: string;
description: string;
// Potentially other props to pass to SEO, like image, type, etc.
image?: string;
type?: "website" | "article";
publishDate?: Date;
// ... and so on for all SEO props you want to make easily accessible
}
const {
title = "Domdhi - Your Personal AI Hub", // Default site title
description = "Exploring the world of AI - tools, coding, art, and automation.", // Default site description
image,
type,
publishDate
// ...destructure other props
} = Astro.props;
---
<!-- Global Metadata -->
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width" />
<link rel="icon" type="image/svg+xml" href="/favicon.svg" />
{/* ...other global meta tags like theme-color, etc. */}
<!-- SEO Tags: All the magic happens here! -->
<SEO
title={title}
description={description}
image={image}
type={type}
publishDate={publishDate}
{...Astro.props} /* Pass through any other relevant SEO props */
/>
{/* ...other global head elements like analytics scripts (loaded defer/async) */}
SEO Best Practices I’m Sticking To
Building the component is one thing; using it effectively is another. Here are some best practices I try to follow:
- Unique Titles & Descriptions: Every page gets a unique, compelling title (under 60 chars) and description (under 160 chars).
- Canonical URLs: Essential for avoiding duplicate content penalties. My component defaults to the current page’s full URL.
- High-Quality OG/Twitter Images: I aim for 1200×630 pixels for clear, engaging social media previews.
- Rich Article Metadata: For blog posts, I always include author, publish/modified dates, and tags. This helps search engines understand the content’s context and freshness.
- Leverage Structured Data: JSON-LD is my friend for potentially getting rich snippets in search results.
- Use
noindex
/nofollow
Sparingly: Only for pages that genuinely shouldn’t be in search results (e.g., draft previews, internal utility pages).
Debugging and Verification
To make sure everything is working as expected, I regularly:
- Inspect Element: A quick check of the
<head>
in browser developer tools. - Use Online Tools: Google’s Rich Results Test, Facebook’s Sharing Debugger, and Twitter’s Card Validator are invaluable.
- Validate Structured Data: Google’s Structured Data Testing Tool (or Schema Markup Validator) helps ensure my JSON-LD is error-free.
Future Enhancements I’m Considering
While the component is quite comprehensive, there’s always room for improvement:
- More granular Open Graph/Twitter Card properties (e.g.,
og:locale
,twitter:site
). - Broader structured data support for different content types beyond articles (e.g., profiles, projects).
- Language-specific metadata (
hreflang
if I ever decide to translate Domdhi.com).
A Foundation for Discoverability
Creating this SEO.astro
component has been a crucial step in building Domdhi.com. It gives me peace of mind knowing that the SEO fundamentals are handled consistently and robustly across the site. It allows me to focus more on creating valuable AI content, knowing that I’ve laid a solid foundation for it to be discovered.
If you’re building a site with Astro (or any framework, really!), I highly recommend investing time in a similar reusable SEO solution. It pays off immensely in the long run!
What are your go-to strategies for SEO in Astro or other frameworks? Any favorite tools or techniques? I’d love to hear about them in the comments!